- Introduction
- Python Execution
- Installing Python Interpreter
- Integrated Development Editor PyCharm IDE
Quick Facts
particular | details | |||
---|---|---|---|---|
Medium of instructions
English
|
Mode of learning
Self study
|
Mode of Delivery
Video and Text Based
|
Course and certificate fees
Fees information
certificate availability
Yes
certificate providing authority
Udemy
The syllabus
Introduction and Python Environment Setup
First Python Program
- Hello world
- Interactive Hello World
- Variables
- Naming Conventions
- Comments in Code
Python Strings
- Python Strings
- type() function
- String operations
- Splitting Strings
- Counting Strings
- Replacing Strings
- Finding Sub Strings
- Converting Other Types into Strings
- Comparing Strings
- Other String Operations
- format() method
Flow control statements
- Comparison Operators
- The If Statement
- Else in an If Statement
- The Use of elif
- Nesting If Statements
Programming Iterative Loops
- While Loop
- For Loop
- For Loop for Strings
- Break Loop Statement
- Continue Loop Statement
- For Loop with Else
Python Functions
- What Are Functions?
- Defining Functions
- Function with Parameter
- Returning Values from Functions
- Returning Values: Two Parameters
- Default Parameter Values
- Named Arguments
- Arbitrary Arguments
- Keyword Arguments
- Anonymous Functions
Python Classes and Objects
- Python Classes
- Class Definitions
- Accessing Object Attributes
- Defining a Default String Representation
- Adding a Behaviour
- Defining Instance Methods
- The del Keyword
- Class Inheritance
Python Properties
- Python Attributes
- Setter and Getter Style Methods
- @Property Decorator
Polymorphism
- Polymorphism
- Polymorphism with Inheritance
Python Modules
- Python Modules
- Importing a Module
- Importing from a Module
- Importing a specific feature
- Importing Within a Function
- Abstract Base Classes (ABCs)
Protocols
- The Context Manager Protocol
- Iterables and Iterators
Decorators in Python
- Defining & Using Decorators
- Decorating Functions with Parameters
- Stacked Decorators
- Parameterised Decorators
- Class Decorators
Python Tuples
- Tuples
- Creating New Tuples from Existing Tuples
- Tuples Can Hold Different Types
- Iterating Over Tuples
- Tuple Related Functions
- Checking if an Element Exists
- Nested Tuples
Python Lists
- Lists
- Nested Lists
- Nested Lists and Tuples
- List Constructor Function
- Accessing Elements from a List
- Adding to a List
- List Concatenation
- Removing from a List
Python Sets
- Sets
- The Set() Constructor Function
- Working with Sets
- Nesting Sets
- Set Operations
Python Dictionaries
- Dictionaries
- Working with Dictionaries
- Iterating over Keys
- Values, Keys and Items
- Checking Key Membership
- Nesting Dictionaries
Python Projects for Beginners
- Dice Roll Game
- Number Guessing Game
- Calculator
- Test your knowledge
Instructors
Articles
Popular Articles
Latest Articles
Similar Courses
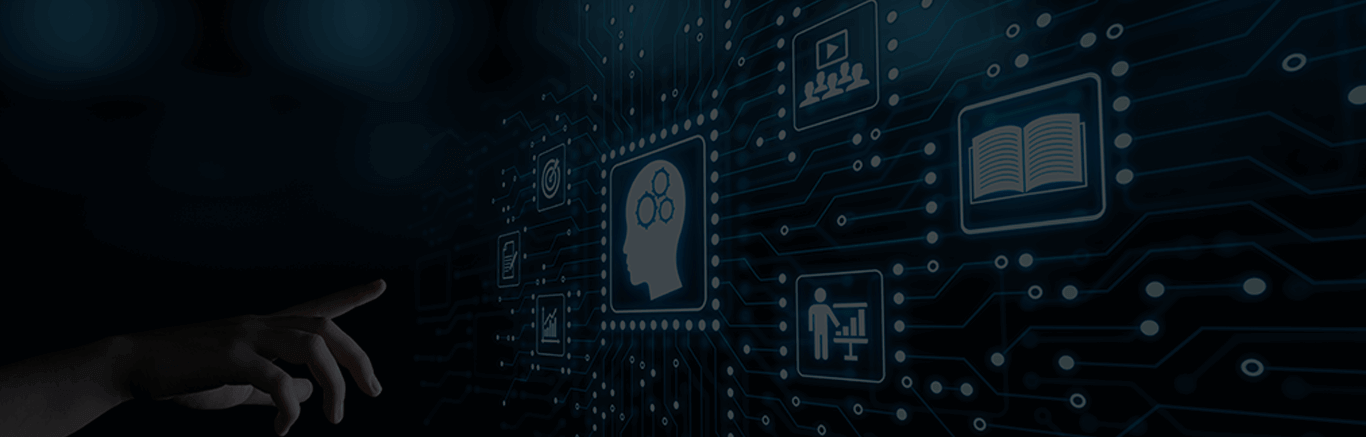
Python Foundations
PW Skills
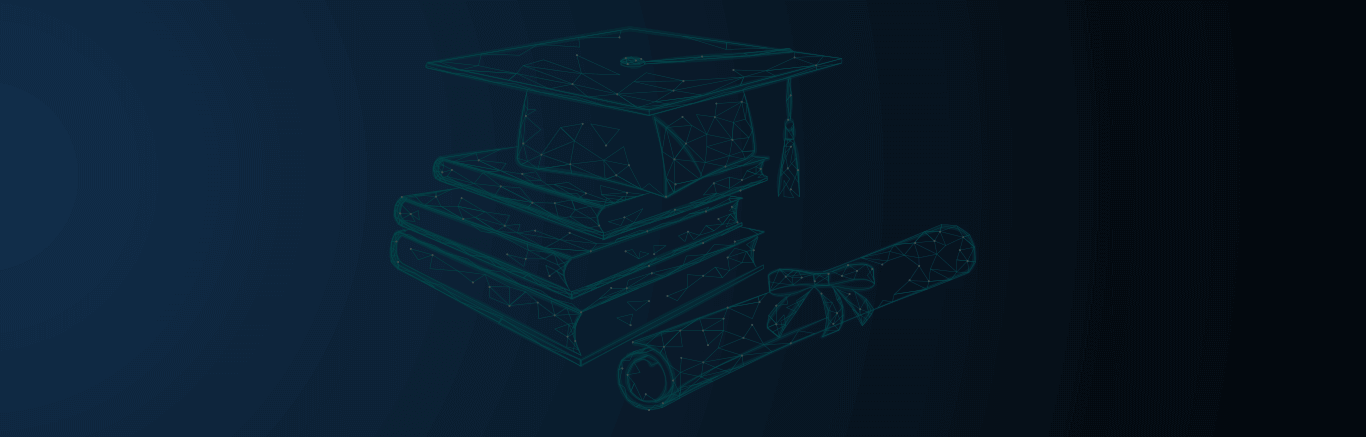
Python Interview Questions and Answers
Great Learning
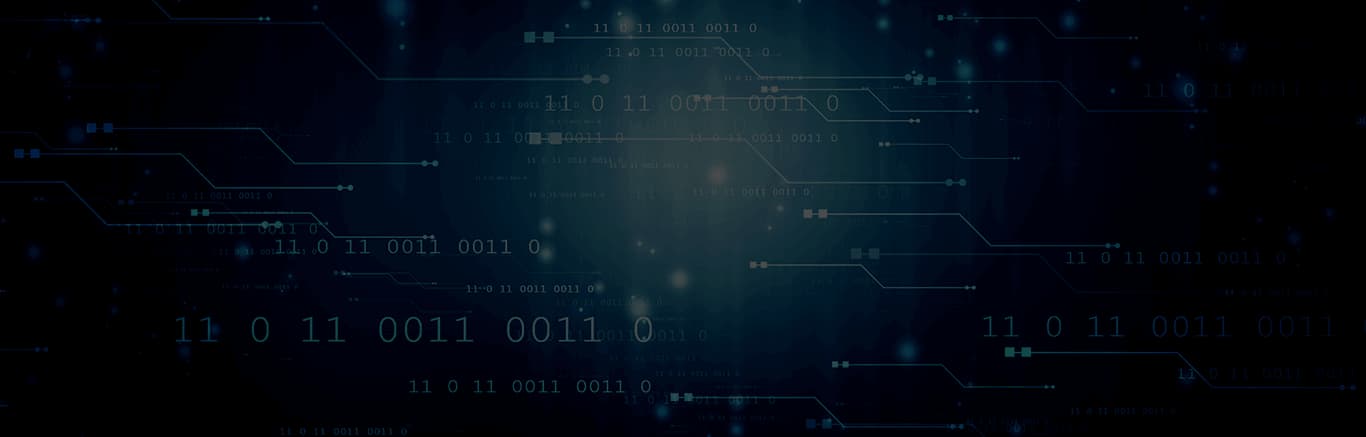
Python Fundamentals for Beginners
Great Learning
Courses of your Interest

Professional Certificate Course in Data Science
Newton School
JavaScript Foundations
PW Skills
Technical Analysis Series
PW Skills
C Programming Foundations
PW Skills

Getting Started with Generative AI APIs
Codio via Coursera

Generating code with ChatGPT API
Codio via Coursera

Prompt Engineering for ChatGPT
Vanderbilt via Coursera
Data Structures and Algorithms in Java
Great Learning